JavaScript DOM Nodes and Navigation..30daysofjavascript ==>Day 19
Nodes in JavaScript is likened to a family tree structure and are defined by hierarchy and measuring from top to bottom or as you may call it, highest to the lowest and consisting of parent, children and siblings. The members of this structure are referred to as nodes. It is worth knowing that a significant characteristic of DOM nodes is that they can be added to the DOM, modified or deleted.
According to the W3C HTML DOM standard, everything in an HTML document is a node, thus elaborating:
- The entire document is a document node
- Every HTML element is an element node
- The text inside HTML elements are text nodes
- Every HTML attribute is an attribute node (deprecated)
- All comments are comment nodes
What is significant in a node relationship?
- The nodes in the node tree have a hierarchical relationship with each other.
- The terms parent, child, and sibling are used to describe relationships.
- In a node tree, the top node is called the root (or root node).
- Every node has exactly one parent, except the root (which has no parent)
- A node can have a number of children.
- Siblings (brothers or sisters) are nodes with the same parent.

From the HTML snippet above you can read:
<html>
is the root node<html>
has no parents<html>
is the parent of<head>
and<body>
<head>
is the first child of<html>
<body>
is the last child of<html>
and:
<head>
has one child:<title>
<title>
has one child (a text node): "DOM Tutorial"<body>
has two children:<h1>
and<p>
<h1>
has one child: "DOM Lesson one"<p>
has one child: "Hello world!"<h1>
and<p>
are siblings
The beauty of JavaScript nodes is the ability to add and remove nodes to/from the node list.
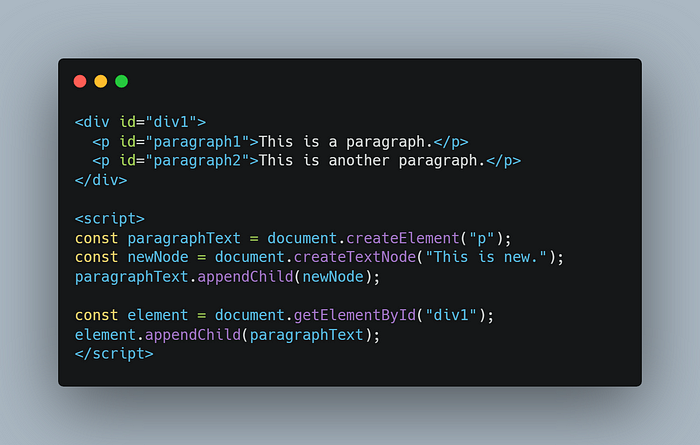
Check here for other examples.
Now, how do we navigate?
Node navigation can be achieved via the following nodes: parentNode, chidNodes, firstChild, lastChild, nextSibling and previousSibling.
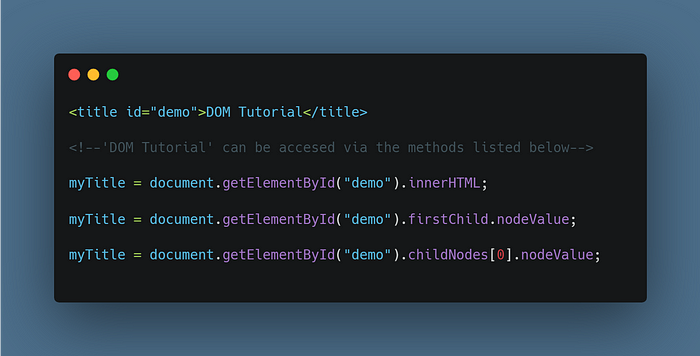
DOM navigation allows content to be copied from one element to another, take a look at the snippets below.



All the snippets above perform the same function of copying the text from the h1 element to the p element.
Asides from the child node and node values that we have looked at above, there are also other methods that are necessary to know in order to navigate in Document Object Model. These methods are named as follows:
DOM Root Nodes
This allows access to the full content of a document using the following methods:
document.body
- The body of the documentdocument.documentElement
- The full document
The nodeName Property
The nodeName
property specifies the name of a node.
- nodeName is read-only
- nodeName of an element node is the same as the tag name
- nodeName of an attribute node is the attribute name
- nodeName of a text node is always #text
- nodeName of the document node is always #document
The nodeValue Property
The nodeValue
property specifies the value of a node.
- nodeValue for element nodes is
null
- nodeValue for text nodes is the text itself
- nodeValue for attribute nodes is the attribute value
The nodeType Property
The nodeType
property is read-only. It returns the type of node.
The above are only theoretical explanations, check here to view live examples at w3schools.
What is this about?
30daysofjavascript is a series of writing on how I learn to code in JavaScript. These episodes are as simplified as possible and for beginners like me, I hope you find JavaScript less confusing throughout this episode. Thank you as always and see you in the next episode. Check out every episode I have written here.